Lightning Component:
<aura:component implements="flexipage:availableForAllPageTypes,force:appHostable">
<!--Attributes-->
<aura:attribute name="showConfirmDialog" type="boolean" default="false"/>
<!--Component Start-->
<div class="slds-m-around_xx-large">
<lightning:button name="delete" variant="brand" label="Delete" onclick="{!c.handleConfirmDialog}"/>
<aura:if isTrue="{!v.showConfirmDialog}">
<!--Modal Box Start-->
<div role="dialog" class="slds-modal slds-fade-in-open ">
<div class="slds-modal__container">
<!--Modal Box Header Start-->
<header class="slds-modal__header">
<h1 class="slds-text-heading--medium">Confirmation</h1>
</header>
<!--Modal Box Header End-->
<!--Modal Box Content Start-->
<div class="slds-modal__content slds-p-around--medium">
<center><b>Are you sure you want to delete this item?</b></center>
</div>
<!--Modal Box Content End-->
<!--Modal Box Button Start-->
<footer class="slds-modal__footer">
<lightning:button name='No' label='No' onclick='{!c.handleConfirmDialogNo}'/>
<lightning:button variant="brand" name='Yes' label='Yes' onclick='{!c.handleConfirmDialogYes}'/>
</footer>
<!--Modal Box Button End-->
</div>
</div>
<div class="slds-backdrop slds-backdrop--open"></div>
</aura:if>
</div>
<!--Component End-->
</aura:component>
Lightning JS Controller:
({
handleConfirmDialog : function(component, event, helper) {
component.set('v.showConfirmDialog', true);
},
handleConfirmDialogYes : function(component, event, helper) {
console.log('Yes');
component.set('v.showConfirmDialog', false);
},
handleConfirmDialogNo : function(component, event, helper) {
console.log('No');
component.set('v.showConfirmDialog', false);
},
})
Output:
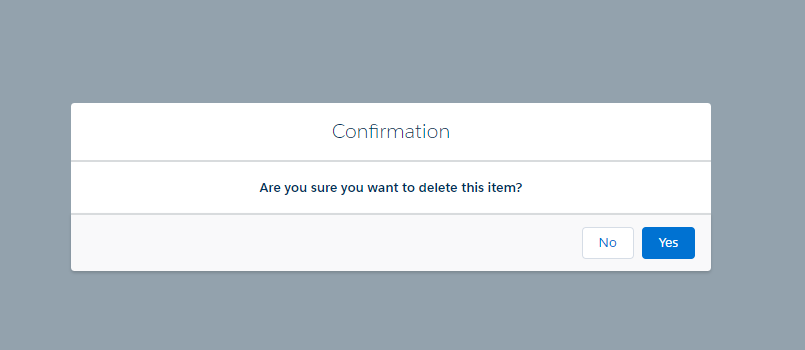
LWCModal.html
<template>
<div class="slds-theme_default">
<div class="slds-p-around_medium">
<lightning-button label="Show LWC Modal" variant="brand" onclick={handleOpenModal}>
</lightning-button>
</div>
<template if:true={isOpenModal}>
<div style="height: 500px;">
<section role="dialog" tabindex="-1" aria-labelledby="modal-heading-01" aria-modal="true" aria-describedby="modal-content-id-1" class="slds-modal slds-fade-in-open">
<div class="slds-modal__container">
<header class="slds-modal__header">
<button class="slds-button slds-button_icon slds-modal__close slds-button_icon-inverse" title="Close" onclick={handleCloseModal}>
<lightning-icon icon-name="utility:close" variant="inverse" alternative-text="Close" size="medium">
</lightning-icon>
<span class="slds-assistive-text">Close</span>
</button>
<h2 id="modal-heading-01" class="slds-text-heading_medium slds-hyphenate">LWC Modal Example</h2>
</header>
<div class="slds-modal__content slds-p-around_medium" id="modal-content-id-1">
<div class="slds-text-heading_small slds-text-align_center">
Welcome to Biswajeet Samal's Blog
</div>
</div>
<footer class="slds-modal__footer">
<lightning-button label="Cancel" variant="neutral" onclick={handleCloseModal}></lightning-button>
</footer>
</div>
</section>
<div class="slds-backdrop slds-backdrop_open"></div>
</div>
</template>
</div>
</template>
LWCModal.js
import {LightningElement, track} from 'lwc';
export default class LWCModal extends LightningElement {
@track isOpenModal = false;
handleOpenModal() {
this.isOpenModal = true;
}
handleCloseModal() {
this.isOpenModal = false;
}
}
LWCModal.js-meta.xml
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata" fqn="LWCModal">
<apiVersion>46.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__AppPage</target>
<target>lightning__RecordPage</target>
<target>lightning__HomePage</target>
</targets>
</LightningComponentBundle>
Output:
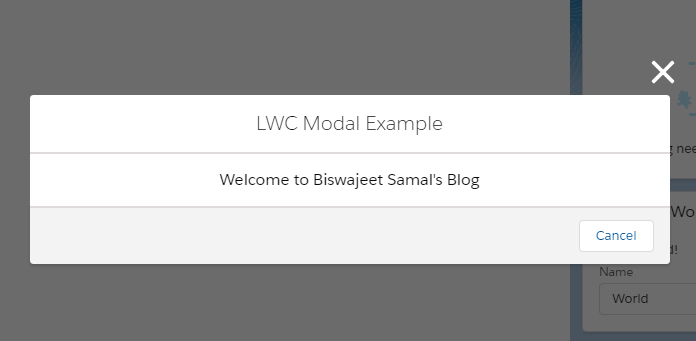
Lightning Component:
<!--demo.cmp-->
<aura:component implements="force:lightningQuickActionWithoutHeader">
<aura:html tag="style">
.cuf-content {
padding: 0 0rem !important;
}
.slds-p-around--medium {
padding: 0rem !important;
}
.slds-modal__content{
overflow-y:hidden !important;
height:unset !important;
max-height:unset !important;
}
</aura:html>
<!--Handler-->
<aura:handler name="init" value="{!this}" action="{!c.doInit}" />
<aura:handler event="aura:doneRendering" action="{!c.doneRendering}"/>
<!--Component Start-->
<div class="slds-m-around--xx-large">
<div class="slds-text-heading_medium slds-align_absolute-center">
Loading...
</div>
</div>
<!--Component End-->
</aura:component>
Lightning JS Controller:
({
doInit : function(component, event, helper) {
//Write your code to execute, before close the quick action modal
},
doneRendering: function(cmp, event, helper) {
$A.get("e.force:closeQuickAction").fire();
}
})
Lightning Component:
<aura:component implements="force:appHostable,flexipage:availableForAllPageTypes,force:lightningQuickAction">
<lightning:button variant="brand" type="button" label="Cancel" title="Cancel" onclick="{!c.handleCancel}"/>
</aura:component>
Lightning JS Controller:
({
handleCancel : function(component, event, helper) {
//to close the quick action modal
$A.get("e.force:closeQuickAction").fire();
},
})