Using lightning:flow
component, we can invoke a flow in Lightning runtime. This component requires API version 41.0 and later. Here is an example to delete all Contact records from an Account using a flow.
Here I’ve created a flow and the flow is invoked by a lightning component, and that lightning component is configured with a QuickAction on Account object.
1. Go to Setup | Quick Find – Search Flows | New Flow
2. Create a variable as “AccountId”
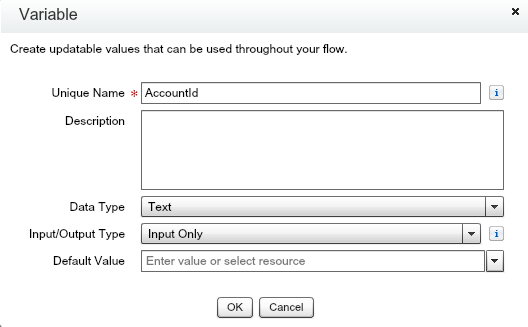
3. Create a sObject Collection Variable as “CollectionOfContacts”
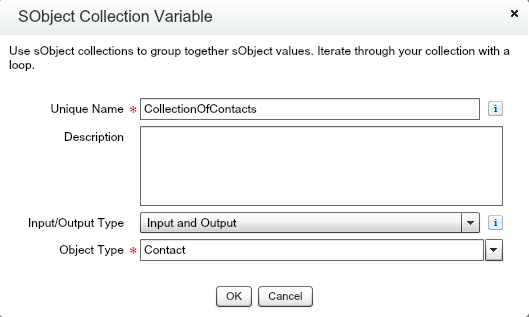
4. Create a Fast Lookup to get Contacts with Account Id
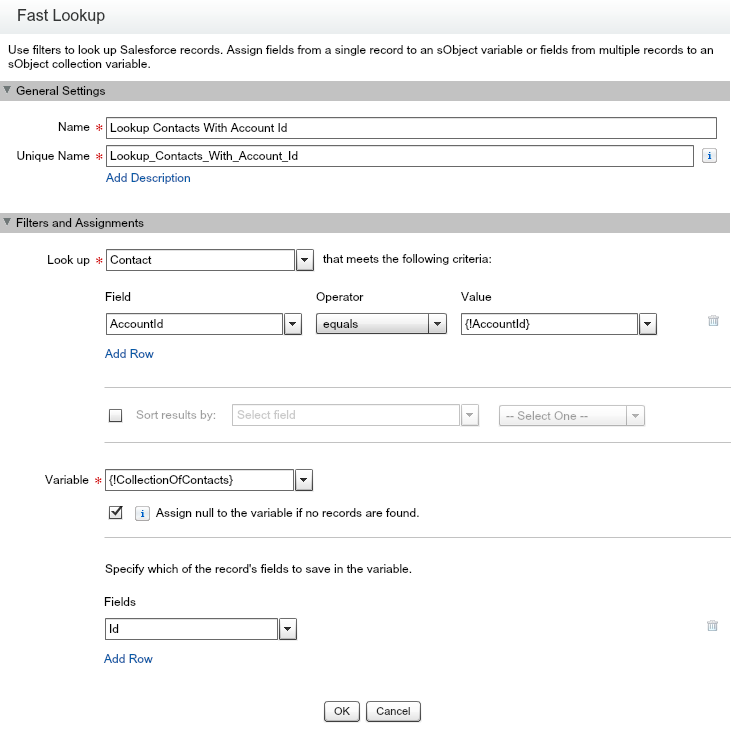
5. Create a Decision to check Account has contacts.
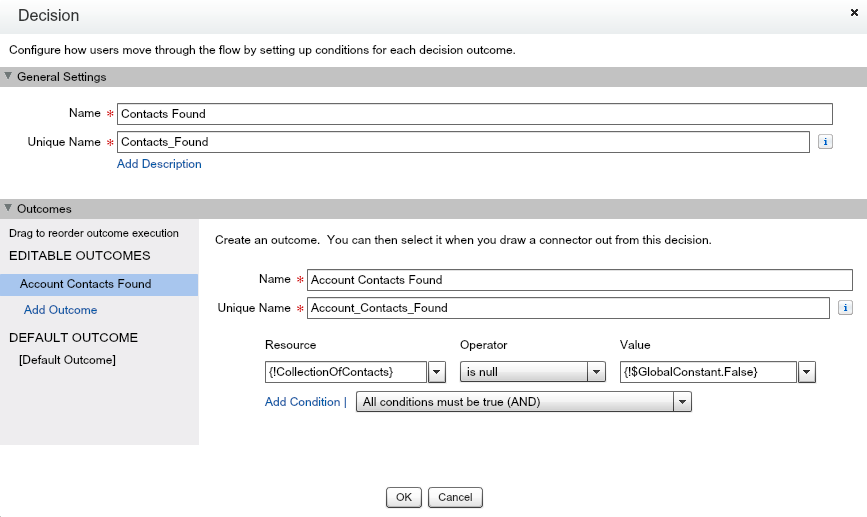
6. Default Outcome in Decision
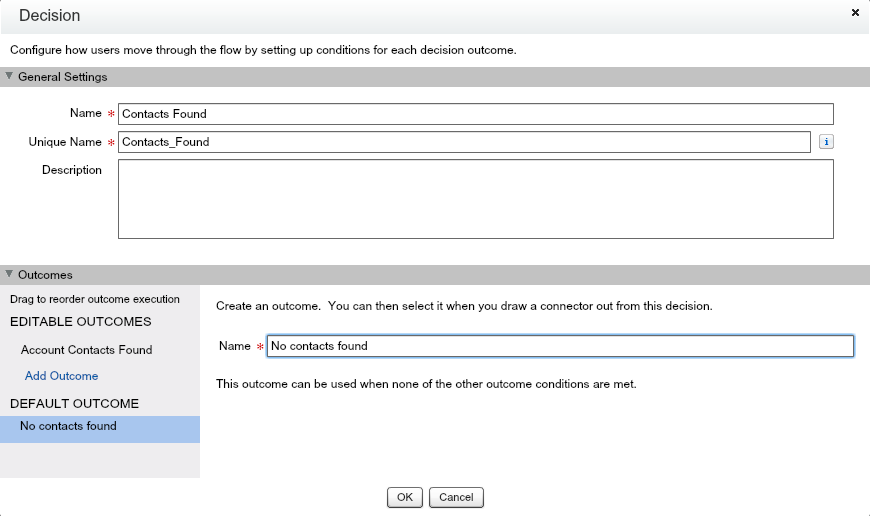
7. Create Fast Delete to delete Contacts
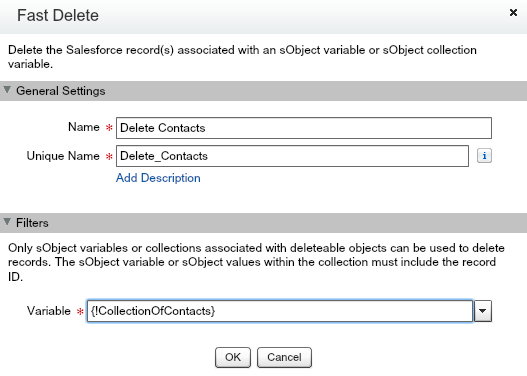
8. Set the Fast Lookup as a starting element, indicated by the green down arrow. Then, connect the flow element “Fast Lookup” to “Decision” and “Decision” to “Fast Delete”.
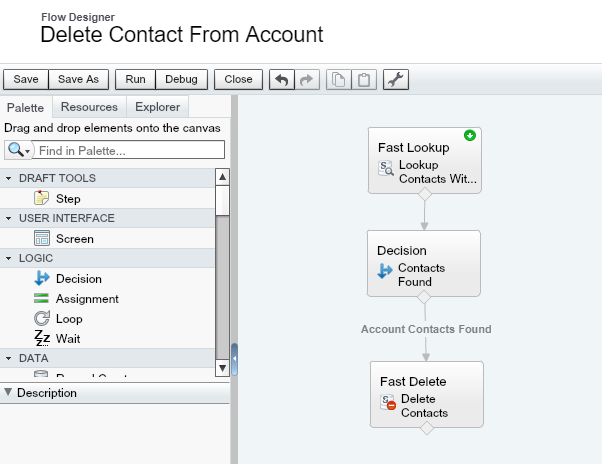
9. Create a Lightning Component to invoke the flow.
Lightning component:
<aura:component implements="force:lightningQuickActionWithoutHeader,flexipage:availableForRecordHome,force:hasRecordId">
<!--Custom Styles for Modal Header and Footer-->
<aura:html tag="style">
.cuf-content {
padding: 0 0rem !important;
}
.slds-p-around--medium {
padding: 0rem !important;
}
.slds-modal__content{
overflow-y:hidden !important;
height:unset !important;
max-height:unset !important;
}
</aura:html>
<!--Declare Attributes-->
<aura:attribute name="hasError" type="Boolean" default="false"/>
<!--Modal Header-->
<div class="modal-header slds-modal__header slds-size_1-of-1">
<h4 class="title slds-text-heading--medium">Delete Contacts</h4>
</div>
<!--End Modal Header-->
<!--Modal Body-->
<div class="slds-modal__content slds-p-around--x-small slds-align_absolute-center slds-size_1-of-1 slds-is-relative">
<form class="slds-form--stacked">
<aura:if isTrue="{!!v.hasError}">
<div class="slds-align_absolute-center">
Do you want to delete all contacts?
</div>
<aura:set attribute="else">
<div>
<div class="slds-text-color_error">Error on Contact record deletion.
Please forward following error message to your admin:</div>
</div>
</aura:set>
</aura:if>
<div>
<!--Lightning Flow Attribute-->
<lightning:flow aura:id="deleteContactFlow" onstatuschange="{!c.statusChange}"/>
</div>
</form>
</div>
<!--End of Modal Body-->
<!--Modal Footer-->
<div class="modal-footer slds-modal__footer slds-size_1-of-1">
<lightning:button variant="Brand" class="slds-button" label="Confirm" onclick="{!c.handleConfirm}"/>
<lightning:button variant="Neutral" class="slds-button" label="Cancel" onclick="{!c.handleClose}"/>
</div>
<!--End of Modal Footer-->
</aura:component>
Lightning JS Controller:
({
//Confirm
handleConfirm : function(component, event, helper) {
//Find lightning flow from component
var flow = component.find("deleteContactFlow");
//Put input variable values
var inputVariables = [
{
name : "AccountId",
type : "String",
value : component.get("v.recordId")
}
];
//Reference flow's Unique Name
flow.startFlow("Delete_Contact_From_Account", inputVariables);
},
//Close the quick action
handleClose : function(component, event, helper) {
$A.get("e.force:closeQuickAction").fire();
},
//Flow Status Change
statusChange : function (component, event, helper) {
//Check Flow Status
if (event.getParam('status') === "FINISHED_SCREEN" || event.getParam('status') === "FINISHED") {
var toastEvent = $A.get("e.force:showToast");
toastEvent.setParams({
title: "Success!",
message: "Contacts deleted successfully!",
type: "success"
});
toastEvent.fire();
$A.get("e.force:closeQuickAction").fire();
$A.get('e.force:refreshView').fire();
} else if (event.getParam('status') === "ERROR") {
component.set("v.hasError", true);
}
}
})
10. Configure above created lightning component on Account object Quick Action.
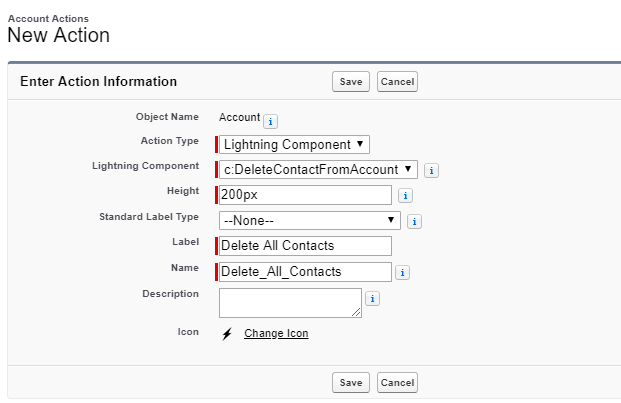
11. Add created quick action on Account object page layout.
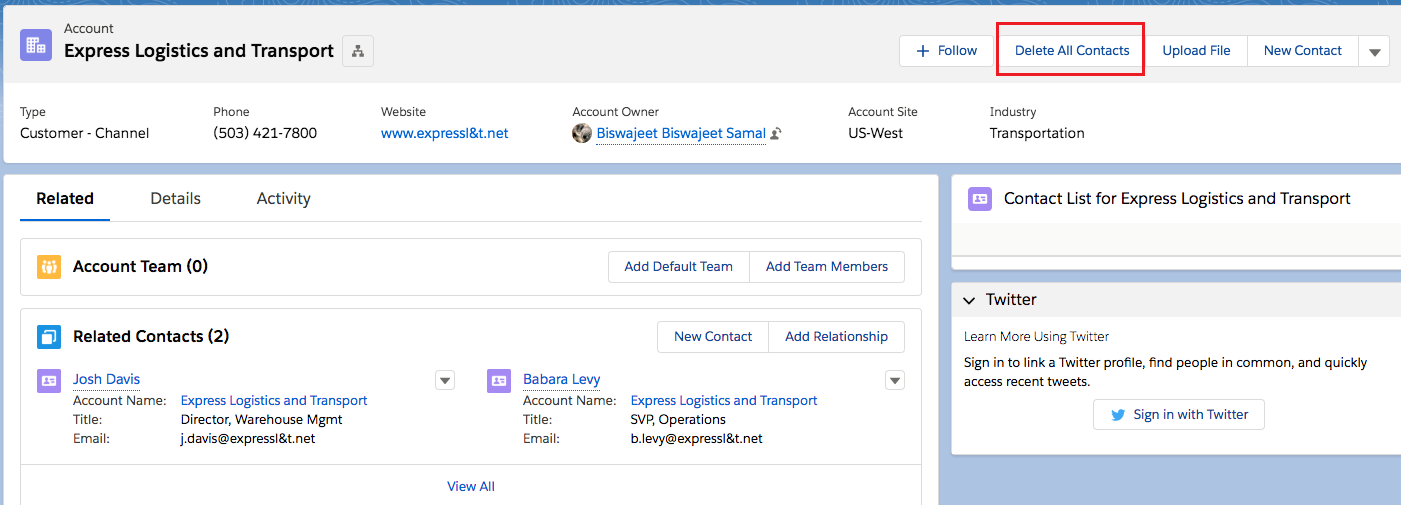
12. Here is the quick action to delete all contacts.
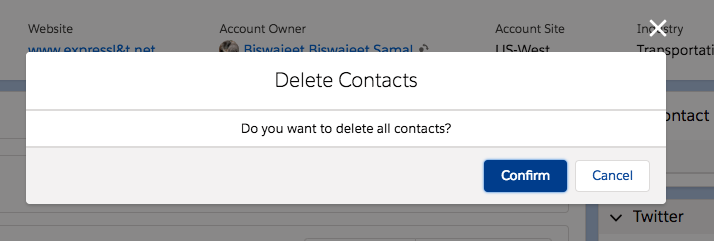