LWCWizard.html
<template>
<lightning-progress-indicator current-step={currentStep} type="base" variant="base">
<lightning-progress-step label="Step 1" value="1" onclick={handleOnStepClick}></lightning-progress-step>
<lightning-progress-step label="Step 2" value="2" onclick={handleOnStepClick}></lightning-progress-step>
<lightning-progress-step label="Step 3" value="3" onclick={handleOnStepClick}></lightning-progress-step>
</lightning-progress-indicator>
<template if:true={isStepOne}>
<div>
Step 1
</div>
</template>
<template if:true={isStepTwo}>
<div>
Step 2
</div>
</template>
<template if:true={isStepThree}>
<div>
Step 3
</div>
</template>
<template if:true={isEnablePrev}>
<lightning-button variant="base" label="Back" onclick={handlePrev}></lightning-button>
</template>
<template if:true={isEnableNext}>
<lightning-button label="Next" variant="brand" onclick={handleNext}></lightning-button>
</template>
<template if:true={isEnableFinish}>
<lightning-button label="Finish" variant="brand" onclick={handleFinish}></lightning-button>
</template>
</template>
LWCWizard.js
import { LightningElement, track} from 'lwc';
export default class LWCWizard extends LightningElement {
@track currentStep = '1';
handleOnStepClick(event) {
this.currentStep = event.target.value;
}
get isStepOne() {
return this.currentStep === "1";
}
get isStepTwo() {
return this.currentStep === "2";
}
get isStepThree() {
return this.currentStep === "3";
}
get isEnableNext() {
return this.currentStep != "3";
}
get isEnablePrev() {
return this.currentStep != "1";
}
get isEnableFinish() {
return this.currentStep === "3";
}
handleNext(){
if(this.currentStep == "1"){
this.currentStep = "2";
}
else if(this.currentStep = "2"){
this.currentStep = "3";
}
}
handlePrev(){
if(this.currentStep == "3"){
this.currentStep = "2";
}
else if(this.currentStep = "2"){
this.currentStep = "1";
}
}
handleFinish(){
}
}
To create “base” style progress indicator you have need to add type="base"
attribute in your lightning-progress-indicator
tag in lightning component.

To create “path” style progress indicator you have need to add type="path"
attribute in your lightning-progress-indicator
tag in lightning component.

Right click on the Lightning Web Component in VS Code | Click on “Delete From Project and Org”.
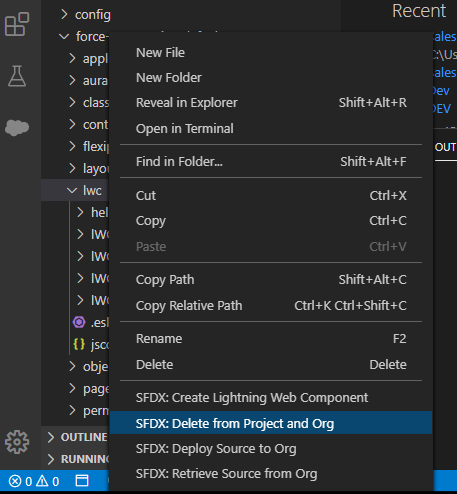
To get current user id in Lightning Web Component, we need to import the "@salesforce/user/Id"
Salesforce scoped module.
LWC.html
<template>
<div class="slds-text-heading_large slds-text-align_center">
User Id : {currentUserId}
</div>
</template>
LWC.js
import { LightningElement } from 'lwc';
import UserId from '@salesforce/user/Id';
export default class UserInformation extends LightningElement {
currentUserId = UserId;
}
Output:

LWCProgressIndicator.html
<template>
<div class="slds-theme_default">
<div class="slds-p-around_medium">
<lightning-progress-indicator type="path" current-step={selectedStep}>
<lightning-progress-step label="Step1" onstepfocus={selectStep1} value="Step1">
</lightning-progress-step>
<lightning-progress-step label="Step2" onstepfocus={selectStep2} value="Step2">
</lightning-progress-step>
<lightning-progress-step label="Step3" onstepfocus={selectStep3} value="Step3">
</lightning-progress-step>
<lightning-progress-step label="Step4" onstepfocus={selectStep4} value="Step4">
</lightning-progress-step>
</lightning-progress-indicator>
<div class="slds-m-vertical_medium">
<lightning-button label="Back" class="slds-m-left_x-small" onclick={handlePrev}></lightning-button>
<template if:false={isSelectStep4}>
<lightning-button variant="brand" class="slds-m-left_x-small" label="Next" onclick={handleNext}></lightning-button>
</template>
<template if:true={isSelectStep4}>
<lightning-button variant="brand" class="slds-m-left_x-small" label="Finish" onclick={handleFinish}></lightning-button>
</template>
</div>
</div>
</div>
</template>
LWCProgressIndicator.js
import { LightningElement, track } from 'lwc';
export default class LWCProgressIndicator extends LightningElement {
@track selectedStep = 'Step1';
handleNext() {
var getselectedStep = this.selectedStep;
if(getselectedStep === 'Step1'){
this.selectedStep = 'Step2';
}
else if(getselectedStep === 'Step2'){
this.selectedStep = 'Step3';
}
else if(getselectedStep === 'Step3'){
this.selectedStep = 'Step4';
}
}
handlePrev() {
var getselectedStep = this.selectedStep;
if(getselectedStep === 'Step2'){
this.selectedStep = 'Step1';
}
else if(getselectedStep === 'Step3'){
this.selectedStep = 'Step2';
}
else if(getselectedStep === 'Step4'){
this.selectedStep = 'Step3';
}
}
handleFinish() {
alert('Finished...');
this.selectedStep = 'Step1';
}
selectStep1() {
this.selectedStep = 'Step1';
}
selectStep2() {
this.selectedStep = 'Step2';
}
selectStep3() {
this.selectedStep = 'Step3';
}
selectStep4() {
this.selectedStep = 'Step4';
}
get isSelectStep4() {
return this.selectedStep === "Step4";
}
}
LWCProgressIndicator.js-meta.xml
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata" fqn="LWCProgressIndicator">
<apiVersion>46.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__AppPage</target>
<target>lightning__RecordPage</target>
<target>lightning__HomePage</target>
</targets>
</LightningComponentBundle>
Output:

LWCProgressBar.html
<template>
<div class="slds-theme_default">
<div class="slds-p-around_medium">
<lightning-progress-bar value={progress}></lightning-progress-bar>
</div>
</div>
</template>
LWCProgressBar.js
import { LightningElement, track } from 'lwc';
export default class LWCProgressBar extends LightningElement {
@track progress = 0;
connectedCallback() {
this._interval = setInterval(() => {
this.progress = this.progress === 100 ? 0 : this.progress + 1;
}, 200);
}
}
LWCProgressBar.js-meta.xml
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata" fqn="LWCProgressBar">
<apiVersion>46.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__AppPage</target>
<target>lightning__RecordPage</target>
<target>lightning__HomePage</target>
</targets>
</LightningComponentBundle>
Output:
